Supercharge Your Python Code with a Comprehensive Testing Strategy
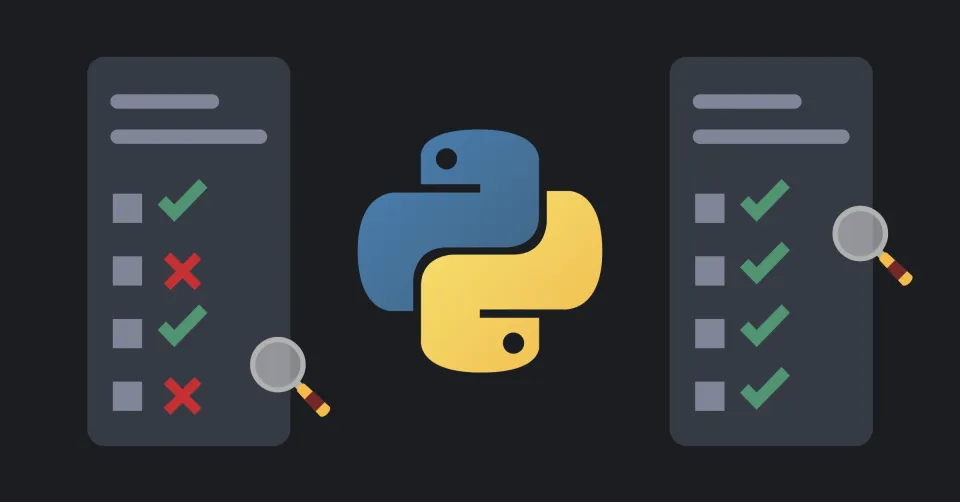
Python is a versatile and widely-used programming language that powers countless applications, from web development to data analysis. To ensure the reliability, security, and maintainability of your Python code, a robust testing strategy is crucial. In this blog post, we'll explore the main steps of a comprehensive Python testing strategy, using tools like Black, Flake8, Bandit, and pytest.
Step 1: Black - Automated Code Formatting
One of the fundamental aspects of code quality is consistent formatting. Black, an opinionated code formatter for Python, automates the process of code formatting. It adheres to PEP 8 guidelines, which are the de facto style guide for Python. By using Black, you can:
- Ensure uniform and clean code formatting.
- Reduce debates over code style within your team.
- Simplify code reviews and collaboration.
The usage of Black is straightforward. Install it with pip install black
and run it on your codebase with black your_project_directory
. It can also easily integrate with various integrated development environments (IDEs) and text editors.
Step 2: Flake8 - Linting and Code Style Checking
Flake8 is a popular Python linting tool that goes beyond formatting and checks your code for adherence to PEP 8 conventions. Using Flake8 allows you to:
- Maintain consistent and readable code.
- Identify potential errors and code quality issues early in development.
- Enforce a common coding style across your projects.
Getting started with Flake8 is as simple as running pip install flake8
and then executing flake8 your_project_directory
. You can customize Flake8's configuration to suit your project's specific requirements.
Step 3: Bandit - Security Code Analysis
Security is a paramount concern in software development. Bandit, a security linter for Python, scans your code for potential security issues and vulnerabilities. By incorporating Bandit into your workflow, you can:
- Identify and address security weaknesses early in the development process.
- Prevent common security vulnerabilities and breaches.
- Promote secure coding practices in your projects.
To get started, install Bandit with pip install bandit
and run it with bandit -r your_project_directory
. You can further customize Bandit's configurations to perform specific security checks.
Step 4: pytest - Writing and Running Tests
Testing is an essential part of a solid Python testing strategy. pytest is a powerful and user-friendly testing framework that simplifies writing and running test cases. With pytest, you can:
- Write concise and scalable test cases using its intuitive syntax.
- Support various types of testing, including unit, functional, and integration testing.
- Generate detailed test reports and easily readable output.
To begin using pytest, install it with pip install pytest
, write test cases as Python functions using the pytest framework, and run tests with the pytest
command in your project directory.
Folder Structure for pytest
Remember that organizing your project directory effectively plays a critical role in successful testing. Typically, you'd structure your folders like this:
my_project/
├── app/
│ ├── init.py
│ ├── module_1.py
│ ├── module_2.py
├── tests/
│ ├── init.py
│ ├── test_module_1.py
│ ├── test_module_2.py
By following this convention, you keep your main code in the app
directory and your test files in the tests
directory. pytest will automatically discover and run your tests.
Key Takeaways
- Black ensures code consistency and readability.
- Flake8 enforces code style and identifies issues.
- Bandit scans for security vulnerabilities.
- pytest simplifies writing and running tests.
Conclusion
In conclusion, a comprehensive testing strategy is vital for the success of your Python projects. By incorporating Black, Flake8, Bandit, and pytest into your workflow and adopting a well-structured folder layout, you can supercharge your Python code, ensuring its quality, security, and maintainability. Start integrating these tools today and witness the benefits they bring to your Python development journey.